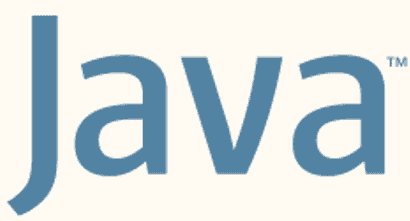
- Published on
Create a Spring Boot Application with Spring Initializr
- Authors
- Name
- Rahul Neelakantan
Download Spring Boot Project from Spring Initializr
Spring Initializr is a web application that allows you to create Spring Boot projects with a few clicks. It is available at https://start.spring.io/.It is straightforward to use. You need to select the project type, the language, the Spring Boot version, and the dependencies. Then, you can download the project as a zip file. You can also generate a Maven or Gradle project. Most of the time, you will use Maven.
For this tutorial, we will create a Spring Boot project with Spring Web
as a dependency. It will create a simple Spring Boot application with a Hello World
endpoint.
Goto https://start.spring.io/ and select the following options as per the below image.

Maven is our chosen build tool, Java is our selected language, and we use the latest non-snapshot version of Spring Boot, which is currently 3.2.1 in this tutorial. Then we select Spring Web
as a dependency. We have the option to choose different dependencies based on our needs, but in this tutorial, we'll stick to Spring Web
.
After selecting the options, click on the Generate
button. It will download a zip file. Extract the zip file and open it in your favorite IDE.
Run Spring Boot Application
Running a Spring Boot application is straightforward. Run it using the IDE or command line. We will use the command line to run the application.
Open the terminal and go to the project directory. Then run the following command.
mvn install
mvn spring-boot:run
The first command will download all the dependencies and build the project. The second command will run the spring boot application. It will start the application on port 8080. You can access the application at http://localhost:8080.
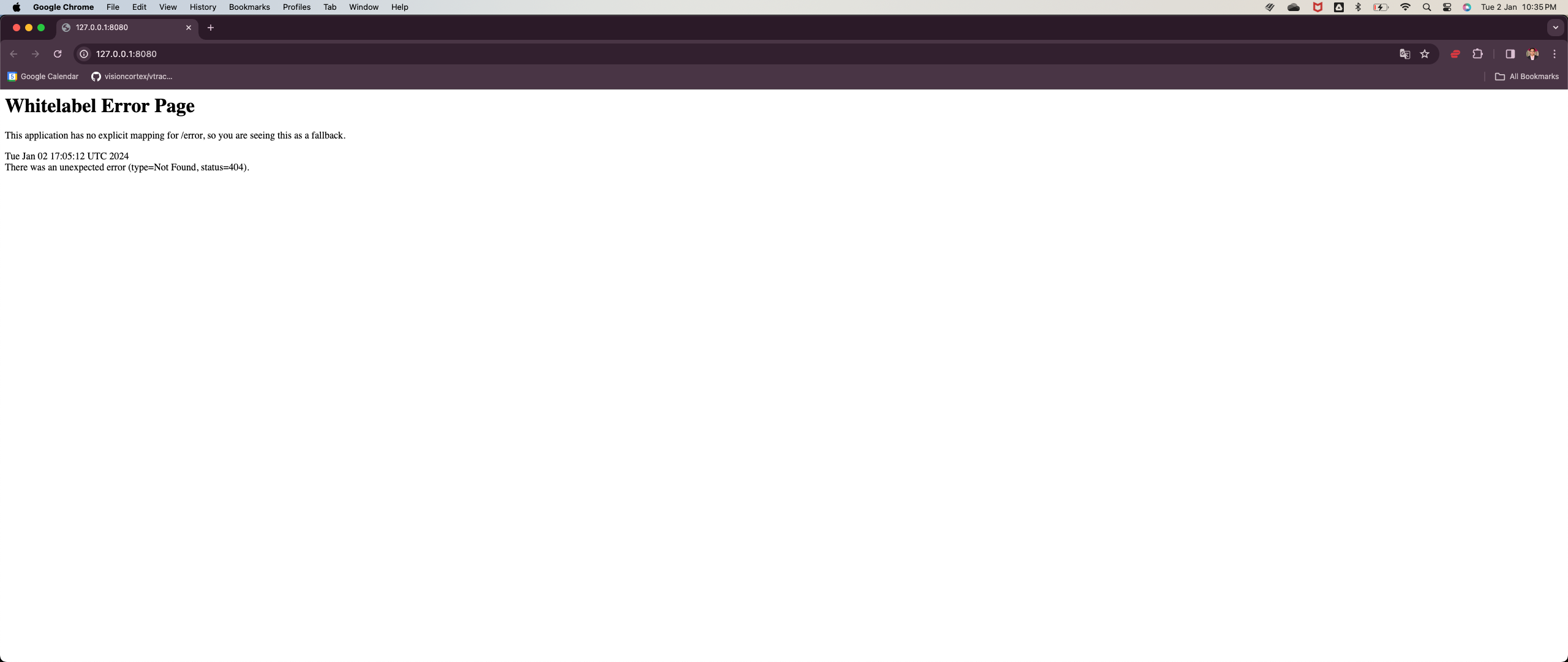
You will get a Whitelabel Error Page
because we have not created any endpoint yet. We will create a simple endpoint that returns Hello World
.
Create a Hello World Endpoint
Create a new class HelloWorldController
in the com.codingjump.helloworld
package. Add the following code to the class.
package com.codingjump.helloworld;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloWorldController {
@GetMapping("/")
public String helloWorld() {
return "Hello World";
}
}
Now run the application again using mvn spring-boot:run
command. When you access the application at http://localhost:8080, you will get Hello World
as a response.
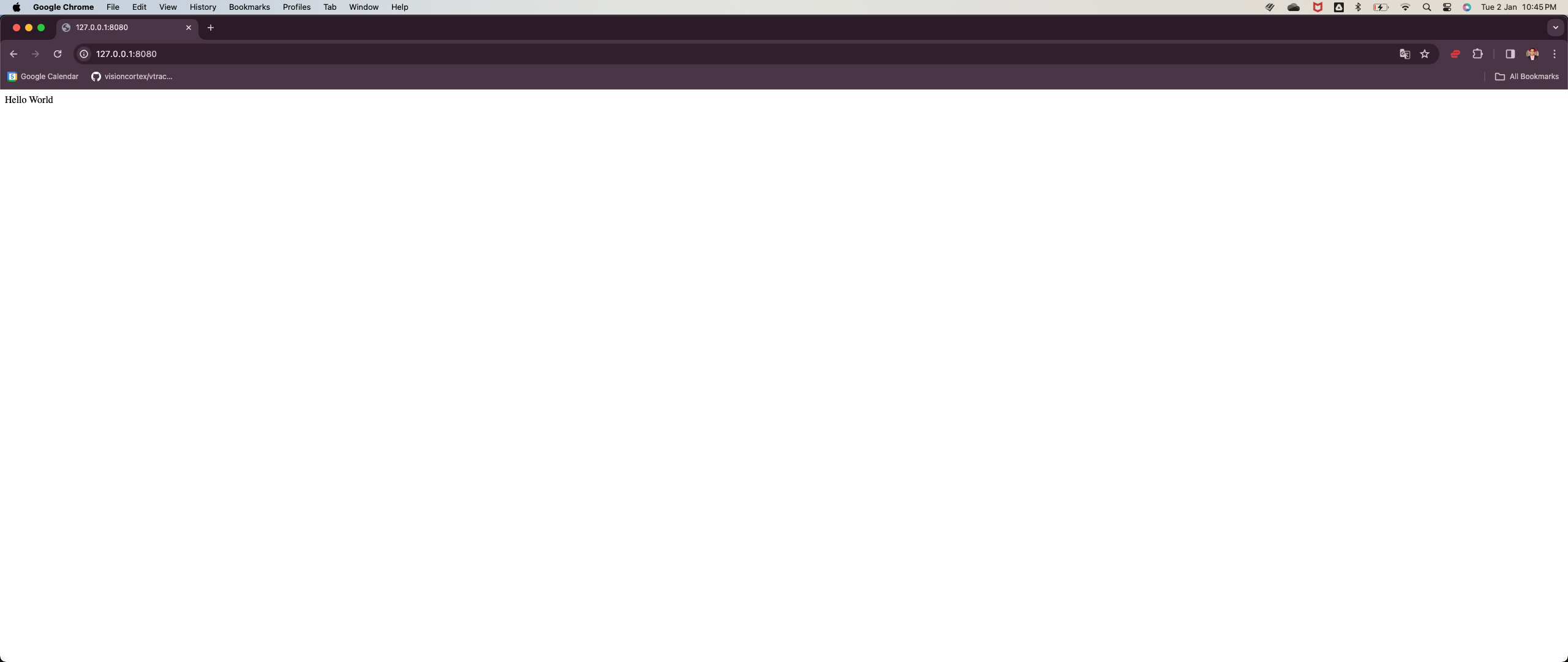
Issues with downloading the dependencies from Maven Central
If you are facing issues with downloading the dependencies from Maven Central, then make sure you turn off the firewall. The firewall could prevent the connection to Maven Central. If that is the case, then you'll need to add an exception to the firewall.
> mvn install
[INFO] Scanning for projects...
Downloading from central: https://repo.maven.apache.org/maven2/org/springframework/boot/spring-boot-starter-parent/3.2.1/spring-boot-starter-parent-3.2.1.pom
[ERROR] [ERROR] Some problems were encountered while processing the POMs:
[FATAL] Non-resolvable parent POM for com.codingjump:helloworld:0.0.1-SNAPSHOT: The following artifacts could not be resolved: org.springframework.boot:spring-boot-starter-parent:pom:3.2.1 (absent): Could not transfer artifact org.springframework.boot:spring-boot-starter-parent:pom:3.2.1 from/to central (https://repo.maven.apache.org/maven2): Broken pipe and 'parent.relativePath' points at no local POM @ line 5, column 10
@
[ERROR] The build could not read 1 project -> [Help 1]
[ERROR]
[ERROR] The project com.codingjump:helloworld:0.0.1-SNAPSHOT (/Users/snrahul11/Downloads/helloworld/pom.xml) has 1 error
[ERROR] Non-resolvable parent POM for com.codingjump:helloworld:0.0.1-SNAPSHOT: The following artifacts could not be resolved: org.springframework.boot:spring-boot-starter-parent:pom:3.2.1 (absent): Could not transfer artifact org.springframework.boot:spring-boot-starter-parent:pom:3.2.1 from/to central (https://repo.maven.apache.org/maven2): Broken pipe and 'parent.relativePath' points at no local POM @ line 5, column 10 -> [Help 2]
[ERROR]
[ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch.
[ERROR] Re-run Maven using the -X switch to enable full debug logging.
[ERROR]
[ERROR] For more information about the errors and possible solutions, please read the following articles:
[ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/ProjectBuildingException
[ERROR] [Help 2] http://cwiki.apache.org/confluence/display/MAVEN/UnresolvableModelException
Conclusion
In this tutorial, we learned how to create a Spring Boot application with Spring Initializr and run it using maven. We also created a simple endpoint that returns Hello World
. You can find the complete code on GitHub.