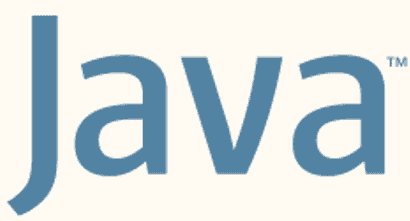
- Published on
Brief Introduction to Java
- Authors
- Name
- Rahul Neelakantan
Java is a general-purpose, class-based, object-oriented programming language. It is fast, secure, and reliable and is platform-agnostic, so it is widely used for developing applications in laptops, data centers, game consoles, scientific supercomputers, cell phones, etc.
Table of Contents
What is Java?
Java was created at Sun Microsystems, Inc., where James Gosling led a team of researchers to develop a new language that would allow consumer electronic devices to communicate with each other. Work on the language began in 1991, and before long, the team’s focus changed to a new niche, the World Wide Web.
Java was first released in 1995, and Java’s ability to provide interactivity and multimedia showed that it was particularly well suited for the Web.
In particular, Java was well-received by developers in the software community because it was created based on the “Write Once, Run Anywhere” (WORA) philosophy.
The Java Runtime Environment, or JRE, is a software layer that runs on top of a computer’s operating system software and provides the class libraries and other resources that a specific Java program needs to run.
Java Runtime Environment is what enables a Java program to run in any operating system without modification.
Although Java 10 and 11 have become popular, Java 8 is still the most used version.
Java Compilers
Java currently has two major variants of Java Compilers:
Oracle Java SE
It is free for development or testing purposes, but you’ll need to take Java SE Subscription for commercial ventures.
Oracle has initially released Java in 1995 and has been continuously working on it to date. Today, Oracle is in charge of Java’s development, despite others like IBM, Red Hat, etc.
Since Java SE 10, we can expect new releases every six months. However, not all releases will be the Long-Term-Support (LTS) releases. As a result of Oracle’s release plan, the LTS product releases will happen only every three years.
Version | Release date |
JDK Beta | 1995 |
JDK 1.0 | Jan-96 |
JDK 1.1 | Feb-97 |
J2SE 1.2 | Dec-98 |
J2SE 1.3 | May-00 |
J2SE 1.4 | Feb-02 |
J2SE 5.0 | Sep-04 |
Java SE 6 | Dec-06 |
Java SE 7 | Jul-11 |
Java SE 8 (LTS) | Mar-14 |
Java SE 9 | Sep-17 |
Java SE 10 | Mar-18 |
Java SE 11 (LTS) | Sep-18 |
Java SE 12 | Mar-19 |
Java SE 13 | Sep-19 |
Java SE 14 | Mar-20 |
Java SE 15 | Sep-20 |
Java SE 16 | Mar-21 |
Java SE 17 (LTS) | Sep-21 |
Java SE 17 is the latest LTS version released, and Java SE 8 will be receiving free public updates until December 2020 for non-commercial usage.
Oracle strongly recommends using the term JDK to refer to the Java SE (Standard Edition) Development Kit.
Oracle JDK was licensed under the Oracle Binary Code License Agreement.
OpenJDK
OpenJDK is a free and open-source implementation of the Java SE Platform Edition. It was initially released in 2007 as the result of the development that Sun Microsystems started in 2006.
Just like for Oracle, the JDK Project will also deliver new feature releases every six months.
OpenJDK has the GNU General Public License (GNU GPL) version 2. and it is increasing in popularity compared to Oracle Java SE.
OpenJDK builds also provided by Oracle. The latest version is JDK16, and JDK17 is in early-access.
OpenJDK and Oracle Java SE keep their version numbers similar, so there is no confusion to developers.
Why is Java still popular?
Java is still the 2nd most popular language below Python. The main reason why Python has gained popularity is due to the popularity of machine learning & artificial intelligence.
Strengths of Java
Automatic Garbage Collection
Java garbage collection is the process by which Java programs perform automatic memory management. Java programs compile to bytecode that can be run on a Java Virtual Machine, or JVM for short. When Java programs run on the JVM, objects are created on the heap, a portion of memory dedicated to the program. Eventually, some objects will no longer be needed. The garbage collector finds these unused objects and deletes them to free up memory.
Garbage collection is automatically done in Java; this saves many headaches for programmers, as they don’t need to keep track of memory and free unnecessary resources manually.
Some compiled languages, such as C, C++, etc., don’t support automatic garbage collection.
Though the garbage collection process in Java is highly fined tuned, the programmer still needs to follow best practices so that the garbage collector works perfectly.
Static Strong Typing Language
Static Typing
A statically typed language has a type system checked at compile time by the implementation (a compiler or interpreter).
Java is a static language; though we can use var in our programs, the datatype is still inferred by the java compiler at compile time. It doesn’t support dynamic typing, so the compiler must know the data type at compile time.
If you’re from a C# background, you might be knowing about dynamic, which Java doesn’t support.
Strong Typing
A strongly typed language doesn’t allow the data type of variable to change at run-time.
Java is a strongly typed language, i.e., say you’ve declared a variable as int, you cannot ask Java to move a String to that variable. Once a variable has been declared as some data type, it cannot be changed till its scope ends.
As Java is a Static, Strongly typed language, programmers are less confused as they don’t need to keep track of data types, etc.
Portability
Java is a programming language that we can use to produce portable software. When we create a Java application, the compiled code (known as bytecode) runs on most operating systems (OS), including Windows, Linux, and Mac OS.
Portability is made possible because of Java Virtual Machine (JVM), which is the layer on which our Java programs execute. JVM takes care of all the machine-specific operations, thereby making Java portable.
JVM is installed when you install Java Runtime Environment (JRE), which is not portable like Java, but it’s just a one-time installation.
Market Share of Java?
Java is not exactly a new language. The year 2020 marks the 25th anniversary of this versatile programming language!
Over the years, Java has maintained its position among the top three most popular programming languages globally, and rightly so. The primary development tool for Android applications is still Java.
Java is used by 3.4% of all the websites whose server-side programming language we know.
Some popular sites using Java are:
Job opportunities for Java developers
Java is the most widely used programming language. There is no denying Java’s popularity with more than nine million developers on seven billion devices worldwide there is no denying Java’s popularity.
Having Java skills is an excellent way to land a job as many companies would anyhow have some project which uses Java as the programming language.
This year Android has a market share of 71.9% of the mobile market. The base language through which we can develop Android applications in Android is Java, so if you’re targeting mobile apps, Java is the way to go.
Also, Java is more popular than C# in terms of Job listings Because if you search Indeed.com, you generally will find more Java than C# jobs.
Popular Libraries
As Java has been there for more than 25 years, many popular open-source projects have been written on it, not to mention the private libraries each company has written over the years.
Below are a few important libraries that every Java developer must know.
Spring Boot
Spring Boot is an open-source Java-based framework used to create a microservice. It is developed by the Pivotal Team and is used to build stand-alone and production-ready spring applications.
It provides a flexible way to configure Java Beans, XML configurations, and Database Transactions.
Spring Boot provides powerful batch processing and manages REST endpoints.
In Spring Boot, everything is auto-configured; no manual configurations are needed.
It offers an annotation-based spring application
Eases dependency management
It includes Embedded Servlet Container
Mockito
Mockito is a mocking framework. It lets you write tests with a clean and simple API. Some advantages of Mockito are it has good community support in the StackOverflow community.
Mockito is present in the Top 10 Java library across all libraries, not only the testing tools.
Hibernate
Hibernate is a high-performance Object/Relational persistence and query service licensed under the open-source GNU Lesser General Public License (LGPL) and is free to download. It takes care of the mapping from Java classes to database tables (and from Java data types to SQL data types) and provides data query and retrieval facilities.
JUnit
The JUnit Platform serves as a foundation for launching testing frameworks on the JVM. It also defines the TestEngine API for developing a testing framework that runs on the platform.
JUnit 5 requires Java 8 (or higher) at runtime. This would be the most downloaded library for Java, as almost every big project would be using it.
Maven
Maven is a software project management and comprehension tool. Based on the project object model (POM) concept, Maven can manage a project’s build, reporting, and documentation from a central piece of information.
Maven is a part of the Apache Software Foundation.
Gradle
Gradle is an open-source build automation tool focused on flexibility and performance. It build scripts are written using Groovy or Kotlin DSL.
There are some fundamental differences in the way that the two systems approach builds. It is based on a graph of task dependencies – in which tasks are the things that do the work – while Maven is based on a fixed and linear model of phases.
What types of applications is Java suitable for?
Mobile Applications with Android
Almost 70% of the mobile phones in the world are Android, so it makes sense that Java is the primary language for building applications for Mobile.
But building applications with Android is a steep learning curve, where we know Java and OS-related stuff, configuring XML properties, etc.
Microservices with Spring Boot
Spring Boot is the leading framework for building microservices applications in Java. With a reported 57% market share, there are plenty of reasons why developers choose Spring Boot for microservices applications.
Spring Boot follows the “opinionated configuration” philosophy, making it super-easy to start with a default auto-wired application template.
Desktop Application with Java
Desktop applications can be quickly developed using Java. We use APIs like AWT, Swing, JavaFX to build these applications.
JavaFX is a modern way to develop a desktop application in Java, and it is graph-based and different from AWT and Swings.
Examples of desktop GUI applications are Acrobat Reader, ThinkFree, Media Player, Antiviruses, etc.
Scientific Applications with Java
Java becomes the best choice for writing scientific applications involving scientific calculations and mathematical operations. It provides a fast, secure, and highly portable environment to these applications, an essential requirement.
MATLAB (Mathematical Laboratory), one of the most famous scientific applications, uses Java to develop both front-end and back-end.
Embedded Systems with JavaME
Embedded systems are present everywhere.
Java shows how efficient its platform is, for which there is a need of just 130 KBs to use on smart cards or sensors.
SIM (Subscriber Identity Module) cards in our phones have been running a variant of the JVM (Java Card) for nearly 20 years.
Other devices like BlueRay Disc players, utility meters, and televisions use Java technology. According to Oracle Corporation, “100% of Blu-ray Disc Players and 125 million TV devices use Java”.
Disadvantages of Java
Performance
Java programs take much longer to run compared to compile languages like C/C++. This is due to having one more additional layer, JRE, which interprets and compiles java programs just before execution. Even though there are so many optimizations done, it still can’t beat the most optimized C/C++ code.
Memory
Since Java programs run on top of Java Virtual Machine, it consumes more memory. Also, as Garbage collection also is automated, if we don’t release the resources, there are higher possibilities of Memory Leak with bad programming practices.
Other Disadvantages
As Garbage collection is done automatically, the programmer has no control over how the memory gets freed, etc. Also, it doesn’t support unsigned types like unsigned int, unsigned char, etc.
There is also no support for low-level programming, like pointers, etc.
Conclusion
I hope this post has given you a sound introduction to Java and its ecosystem. We have just scratched the surface of what Java is and what it can do for us?
Java has two main components JRE and JDK, and every system needs to have JRE installed for it to run Java applications. JDK is primarily used for developing Java applications.