
- Published on
How to manage dependencies for your .Net Projects?
- Authors
- Name
- Rahul Neelakantan
Any long running project will have numerous opensource dependencies. Adding these dependencies is made very easy with Nuget packages, etc. This article will give you a gist of details on how you can go about managing your dependencies for project.
What is a Nuget package?
Put simply, a NuGet package is a single ZIP file with the .nupkg extension that contains compiled code (DLLs), other files related to that code, and a descriptive manifest that includes information like the package’s version number. Developers with code to share create packages and publish them to a public or private host. Package consumers obtain those packages from suitable hosts, add them to their projects, and then call a package’s functionality in their project code. NuGet itself then handles all the intermediate details.
Nuget package in C# or any Dot.Net Project is very similar to how NPM works in NodeJS.
How to manage Nuget package using Visual Studio?
You can manage Nuget packages very easily with Visual Studio. I’m using Visual Studio 2019 for the below example.
When you right click on a project, you’ll find a menu appearing, where you can select Manage NuGet Packages.
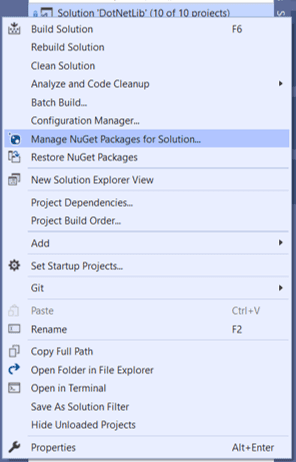
Click on that then you’ll find another window opening where you’ll find several tabs Browse, Installed & Updates.
- Browse can be used for searching over nuget.org & other Nuget feeds which you can install for your project.
- Installed displays all the packages that are installed for the project, you can update/uninstall the Nuget package from here.
- Updates displays all the packages which are older i.e., they have latest packages available for upgrade.
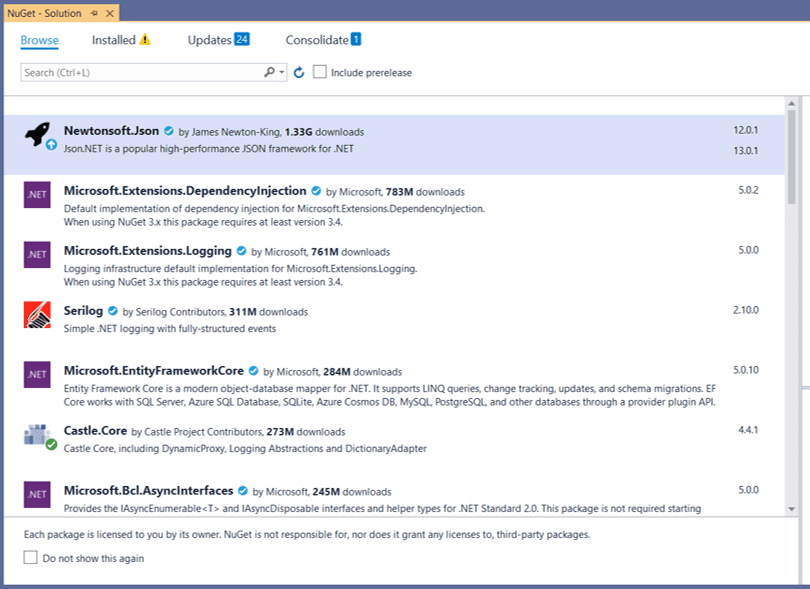
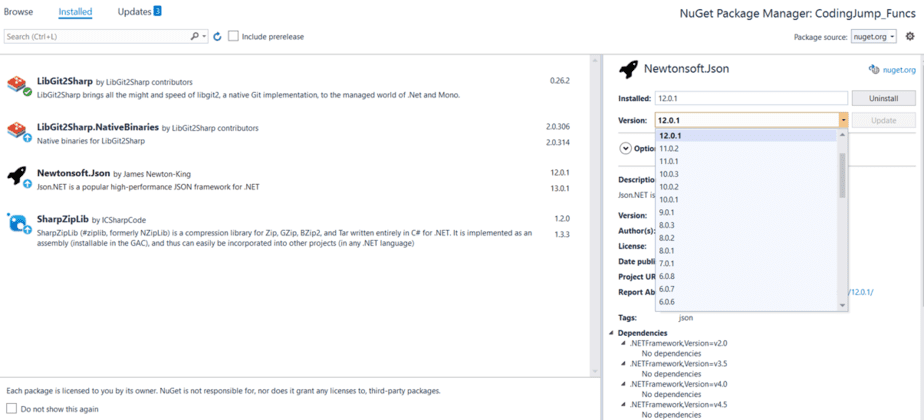
How to manage Nuget package using nuget.exe?
You can download nuget.exe & add this exe to your path. Once you do the same you can then use this directly for updating you Nuget packages from command line. Let’s assume that you need to update EntityFramework for your project then you can run the below command. For update you provide the VS project file.
nuget update -i EntityFramework CodingJump_SQLiteDB\CodingJump_SQLiteDB.proj
Similarly we can install new packages using below command.
nuget install EntityFramework -o CodingJump_SQLiteDB
Nuget command line has many other options which you can explore in the official documentation.
Why do we need to update our Nuget packages?
Nuget packages are libraries that are being maintained by other developers. Which can be continuously updated to build new features, resolve existing bugs, etc.
If we don’t update our packages frequently then we might be depending on Nuget packages that are not supported by the original developers, then if any new bugs we encountered might have been already fixed in the latest versions.
Updating our packages frequently is more suggested rather than updating it after many years as there may be massive number of API changes that we must resolve first. A single twig breaks, but the bundle of twigs is strong.
What if you have numerous packages installed in your solution?
Each project maintains its own packages.config, whereit stores all the package information. If a project A is dependent on another Project B in a solution, then it will inherit all the Nuget packages from B along with its own.
If you want to update any Nuget package version, you’ll have to do so for each project present in the solution. Doing so would make the process very tedious even if you do it from the command line or batch file.
We have a perfect solution for the same i.e., NuKeeper to the rescue. This takes care of automatically updating your Nuget package for your entire Solution i.e., all Projects will be updated with the required Nuget package.
Working with NuKeeper
NuKeeper is an opensource project which works with both .Net Framework & .Net Core. Here is the GitHub repository for the same, where you can read more about it. In this section I’ll be introducing some of the features of NuKeeper on how you can mange your dependencies efficiently. Before updating our Nuget packages we’ll need to inspect our Nuget package versions & how old they are from what’s available for those dependencies now.
> nukeeper inspect
Found 65 packages
Found 65 packages in use, 32 distinct, in 8 projects.
Castle.Core, cef.redist.x64, cef.redist.x86, CefSharp.Common, CefSharp.WinForms, ClosedXML, CommandLineParser, ConsoleProgressBar, CS-Script.lib, DocumentFormat.OpenXml, EntityFramework, ExcelNumberFormat, FastMember, FastMember.Signed, Ionic.Zip, Jint, LibGit2Sharp, LibGit2Sharp.NativeBinaries, LiteDB, Microsoft.Office.Interop.Excel, Newtonsoft.Json, NLog, NUnit, SharpYaml, SharpZipLib, Sprache, System.Data.SQLite, System.Data.SQLite.Core, System.Data.SQLite.EF6, System.Data.SQLite.Linq, System.IO.FileSystem.Primitives, System.IO.Packaging
Found 26 possible updates
...
Found 26 package updates
EntityFramework to 6.4.4 from 6.2.0 in 5 places since 1 year and 5 months ago.
System.Data.SQLite.Core to 1.0.115 from 1.0.111.0 in 5 places since 2 months ago.
System.Data.SQLite.EF6 to 1.0.115 from 1.0.111.0 in 5 places since 2 months ago.
System.Data.SQLite.Linq to 1.0.115 from 1.0.111.0 in 5 places since 2 months ago.
System.Data.SQLite to 1.0.115 from 1.0.111.0 in 5 places since 2 months ago.
...
nukeeper update -i "EntityFramework"
nukeeper update -i "System.Data.SQLite*"
One more point to note is we need to update the Nuget packages one by one as whenever we upgrade there are chances that the API can get deprecated/removed. There may be bugs also with the latest versions, etc. So, after upgrading the package we’ll have to check if everything builds successfully and then proceed with unit & end to end testing.
This approach must be followed for each Nuget package in your project, but I think it would best hold off manual testing after all Nuget packages have been updated. As manual testing is very tedious to do for every upgrade.
Conclusion
If you’re project needs to survive the test of time, it needs to be frequently updated to the latest Nuget packages. If delayed then we may not find the motivation later as there may be lot of changes in every Nuget package, so upgrading might not be feasible without major rework in codebase.
My recommendation would be to update your Nuget packages every 3/6 months, but it all depends on your project timeline & complexity